Synchronize macOS account picture with Entra ID (formerly Azure AD)
Content
- Purpose
- Solution
- Sources / usefull resources
Purpose
One day, I wanted to synchronize Entra ID (formerly Azure AD) account picture with macOS devices account picture, so user will have the same behavior on Windows and macOS. I waited for Entra ID Platform SSO to comes out, but public preview shows that it does not synchronize user picture.
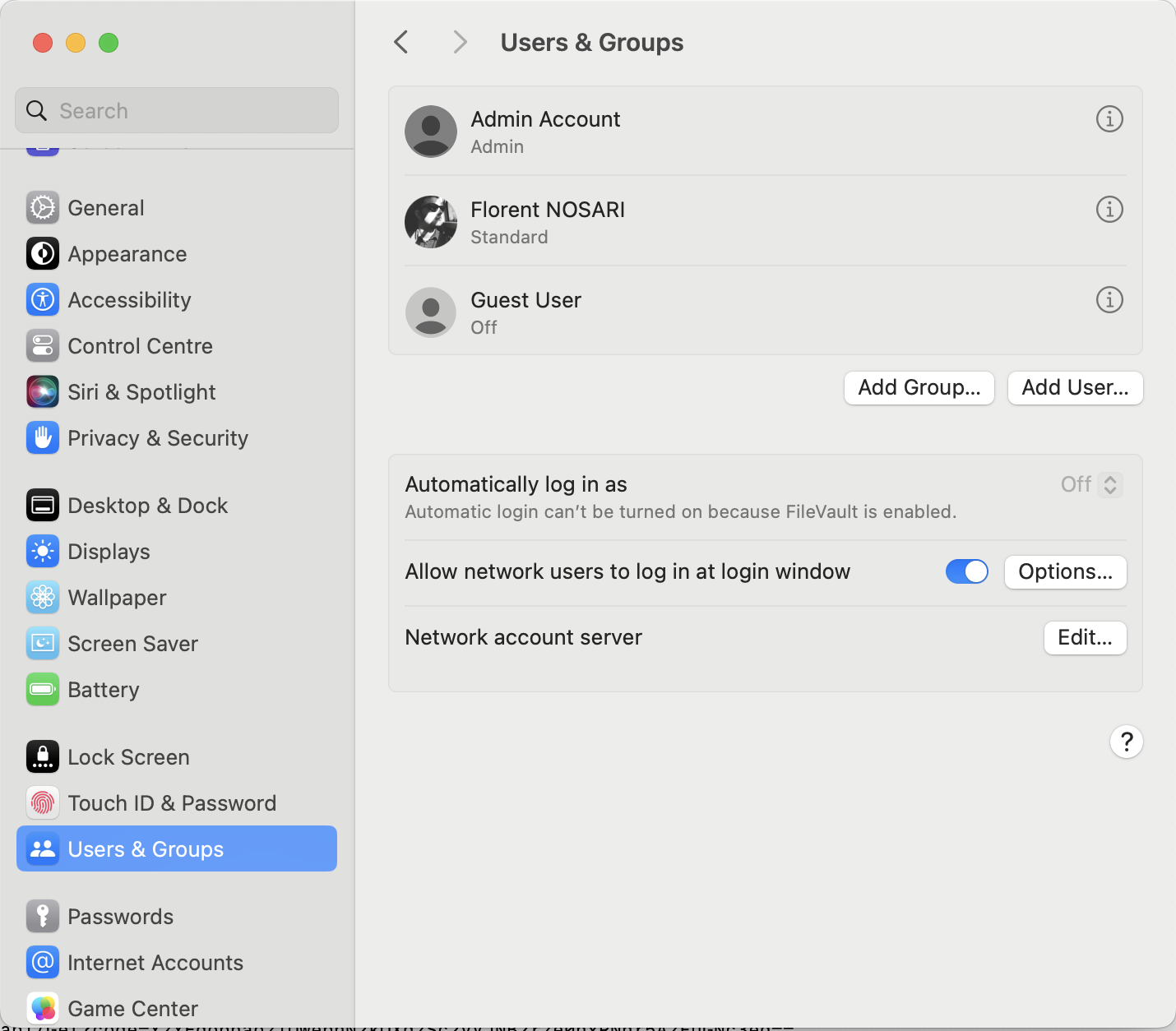
Solution
Here is a quick overview of what my solution looks like:
- Azure Function as midleware which will use MS Graph API to retrieve user picture. I choose to use it to prevent Graph API credentials exposure (see my previous post about this).
- A Shell script, run periodically by MDM, which perform HTTP calls to the Azure Function in order to retrieve user picture and set it as account picture.
Azure Function code
This Azure function uses the same base as the one here and require client certificate (certificate is validated in function code). You have to change line 42 to put the base64 string of your own CA (and sub-CA). Unfortunately you cannot use Intune MDM certificate as it does not allow all apps to use it (do not forget to allow all apps to use the certificate you deploy, I suggest to use a dedicated configuration and put purpose as OU like in my example).
The certificate is checked but you can improve security by adding the following checks:
- Check that device still exist in MDM
- Check that device is compliant
- Put owner UPN in certificate
To simplify requests, I choose to make a unique endpoint and return picture data directly when applicable.
|
|
Shell script
The client script relies on work from copyprogramming.com for setting user picture. I choose to iterate over users accounts to make all users have the proper picture, as I am using Entra ID Platform SSO to create accounts I filter the result to get only user containing domain (@ is ommited during creation but user have to login using UPN as well).
You may have to change line 107 to match what you choose as OU and eventually change the findCertAlias
function if you need more criteria.
Note: the string CURL_SSL_BACKEND=secure_transport
before the curl
command is used in order to allow curl
to use certificates in keychain instead of files.
Note 2: Users will need to reboot devices to apply change on login screen.
|
|